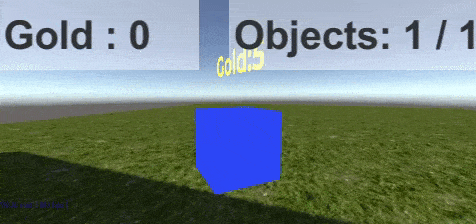
Description
This project represents a simple 3D game prototype. It's an endless game, the player should collect objects on two levels. Each object has its own type and some gold attached to it. Once all objects are collected, new objects are spawned. The game progress can be saved and loaded.
Features
- Very simple project with simple scripts
- Code generation usage example
- Data binders usage example
- Save Load addon usage example
- Text tutorial available (read below)
Setup
- Download zip archive, unzip it and open the project in Unity Editor (version >= 2019 LTS). Ignore error messages
- Import BGDatabase asset
- If you use Unity 2022, you may see compilation errors in the console. Import "Unity UI" package using Unity package manager
- Run example scene (BGDatabaseBasic3DExample\Scenes\BGExample1.unity)
Database structure
Created with Diagram addon
Guides
Read in-depth guides
Description | Link |
---|---|
Database schema | read |
Code generation | read |
SaveLoad addon merge settings. How to use row-level controller to add new scenes after game release | read |
Code explanation | read |
Releases
Click to see all releases
Version | Release date | Log |
---|---|---|
1.3 | Jul 18, 2024 | Custom row level controller is removed in favor of builtin controller |
1.2 | Jul 14, 2024 | Added Version table and row level controller for adding new scenes after game release (read the guide above for more details) |
1.1 | Nov 10, 2021 | Graph binder adn action field examples are added |
1.0 | March 30, 2020 | Initial release |